首先查看程序信息:
1 | Arch: amd64-64-little |
使用IDA打开,main函数:
1 | int __fastcall main(int argc, const char **argv, const char **envp) |
依旧是一个很经典的菜单题。touch()
中表面仅允许创建10个chunk,chunk size最大为0x200。所有chunk指针均存放在全局指针变量buf
中:
1 | unsigned __int64 touch() |
delete()
中将free后的指针置为了NULL,那么很难再UAF。
1 | unsigned __int64 delete() |
take_note()
编辑堆块内容时存在溢出漏洞,可以以此为切入点:
1 | unsigned __int64 take_note() |
远程服务器为Ubuntu 16.04,并且已经提示为unlink漏洞。可以利用该漏洞以及buf
,修改__free_hook
为system
,从而getshell。
exp
1 | from pwn import * |
利用结果:
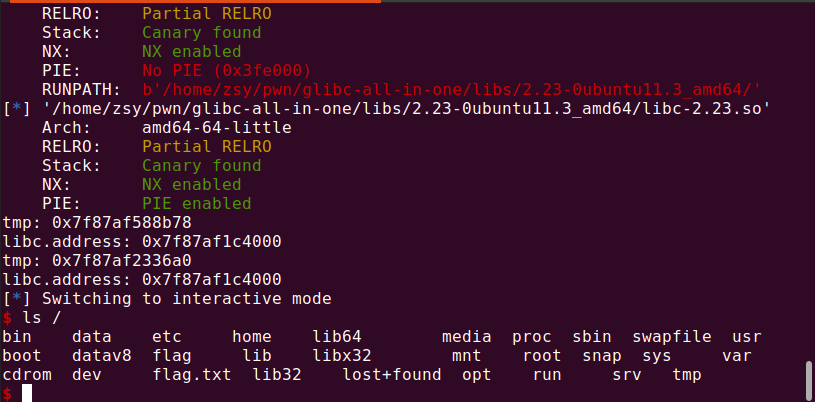